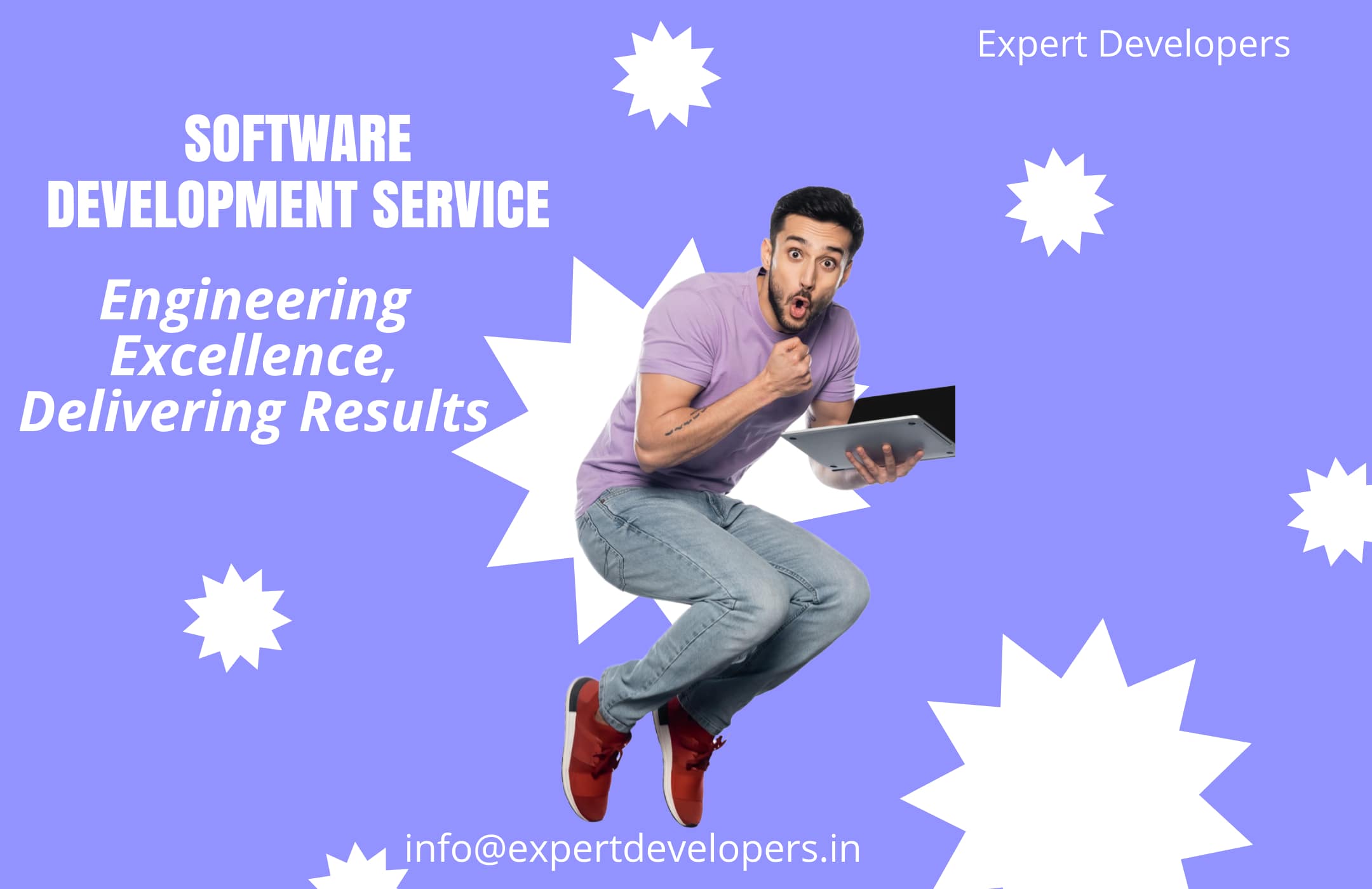
The landscape of backend development is constantly evolving. As we approach 2025, microservices architecture reigns supreme, and Node.js continues to be a powerful contender. This article dives deep into how you can leverage Node.js and serverless functions to build secure and scalable microservices, focusing on best practices for authentication, authorization, and data protection.
Why Node.js for Microservices?
Node.js’s non-blocking, event-driven architecture makes it exceptionally well-suited for handling concurrent requests, a key requirement for microservices. Its lightweight nature and vibrant ecosystem of npm packages allow developers to quickly build and deploy individual services. The shared JavaScript codebase between frontend and backend also streamlines development workflows.
The Serverless Advantage
Serverless functions, such as AWS Lambda, Azure Functions, and Google Cloud Functions, offer several benefits for microservices: automatic scaling, pay-per-execution pricing, and reduced operational overhead. This allows developers to focus on writing code rather than managing infrastructure. By deploying Node.js microservices as serverless functions, you can achieve significant cost savings and improved scalability.
Securing Your Node.js Serverless Microservices
Security is paramount, especially in distributed architectures like microservices. Here's how to secure your Node.js serverless microservices:
- Authentication: Implement robust authentication mechanisms like JSON Web Tokens (JWT). Verify user identities before granting access to resources. Use libraries like `jsonwebtoken` and `passport.js` for simplified JWT management and authentication strategies.
- Authorization: Enforce granular authorization policies using Role-Based Access Control (RBAC) or Attribute-Based Access Control (ABAC). Determine what each user is allowed to do based on their role or specific attributes. Libraries like `casbin` can help implement these policies.
- Input Validation: Sanitize and validate all incoming data to prevent injection attacks (e.g., SQL injection, cross-site scripting). Utilize libraries like `joi` and `validator` for robust input validation.
- Secrets Management: Never hardcode sensitive information like API keys and database passwords. Use secure secrets management services provided by your cloud provider (e.g., AWS Secrets Manager, Azure Key Vault, Google Cloud Secret Manager). Access these secrets from your Node.js functions using environment variables.
- Data Encryption: Encrypt sensitive data both in transit and at rest. Use HTTPS for all communication and encrypt data stored in databases using encryption keys managed securely.
- Rate Limiting: Implement rate limiting to protect your services from abuse and denial-of-service attacks. Cloud providers offer built-in rate limiting capabilities or you can use middleware like `express-rate-limit` in your Node.js functions.
- Vulnerability Scanning: Regularly scan your code and dependencies for known vulnerabilities. Tools like `npm audit` and Snyk can identify and help you fix vulnerabilities.
- Monitoring and Logging: Implement comprehensive monitoring and logging to detect and respond to security incidents. Use centralized logging services like ELK stack or Splunk to analyze logs and identify suspicious activity.
Example: JWT Authentication in a Serverless Function
Here's a simplified example of how to implement JWT authentication in a Node.js serverless function using AWS Lambda and `jsonwebtoken`:
const jwt = require('jsonwebtoken');
exports.handler = async (event) => {
try {
const token = event.headers.Authorization.split(' ')[1];
const decoded = jwt.verify(token, process.env.JWT_SECRET);
// Access user data from the decoded token (e.g., decoded.userId)
console.log('User ID:', decoded.userId);
// Your logic here
return {
statusCode: 200,
body: JSON.stringify({ message: 'Authentication successful' }),
};
} catch (error) {
return {
statusCode: 401,
body: JSON.stringify({ message: 'Authentication failed' }),
};
}
};
Conclusion
Node.js and serverless functions are a powerful combination for building scalable and cost-effective microservices architectures. By prioritizing security at every stage of development and implementation, you can ensure the integrity and confidentiality of your data and protect your applications from threats. As we move towards 2025, embracing these technologies and best practices will be crucial for building robust and secure backend systems.
Comments
Post a Comment